Basic Computer Vision
Goal
Here the intention is to develop some basic exercises about computer vision. You will have to get in contact with the OpenCV (Python) library.
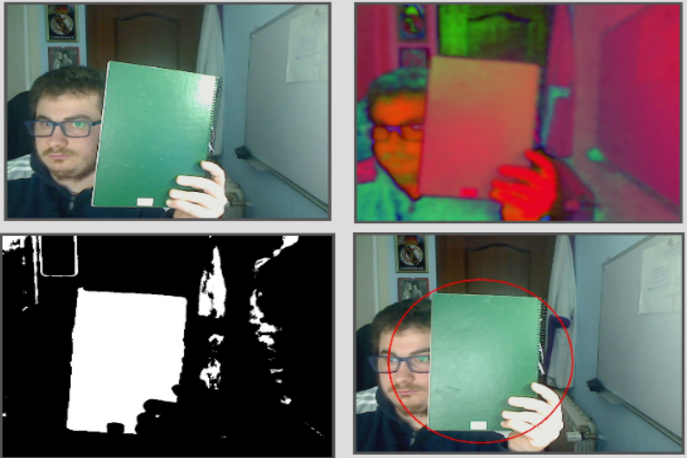
Note: If you haven’t, take a look at the user guide to understand how the installation is done, how to launch a RoboticsBackend and how to access the exercises.
Exercise API
GUI.getImage()
- to get the image. It can be None.GUI.showImage()
- allows you to view a debug image or one with relevant information.
Theory
In this exercise different computer vision functionalities are proposed for their implementation:
- Change to grayscale
- Morphological processing
- Implement a color filter
- Edge filters (for example canny or laplace)
- Convolutions (smoothing and enhancing)
- Optical flow
- Corner detector
- Hough transform
Grayscale
It is proposed to transform the image obtained from the camera into a grayscale image.
Morphological Processing
It is proposed to apply different morphological filters to the image, such as dilation, erosion, etc. Morphological filters are a set of image processing techniques primarily used for binary or high-contrast images. These operations are based on the shape or structure of the objects within an image and are designed to transform the shapes of objects in a way that depends on their structure. Morphological filters are widely used in tasks such as noise removal, filling holes, edge detection, and image segmentation.
Common Morphological Operations:
-
Erosion: Erosion reduces the size of the objects in an image. For each pixel in the image, it checks if all the pixels under the mask (structuring element) are 1 (white). If they are, the central pixel remains white; if not, it turns black. In practice, this operation “shrinks” the white regions in the image.
-
Dilation: Dilation increases the size of the objects in the image. For each pixel in the image, if at least one of the pixels under the mask is 1, the central pixel becomes white. In practice, this operation “expands” the white regions in the image.
-
Opening: The opening operation is a combination of erosion followed by dilation. It removes small objects and fine details from the image.
-
Closing: The morphological closing filter is a combination of two basic morphological operations: dilation followed by erosion. It is used to remove small holes or gaps inside objects, while preserving the overall shape and size of the objects.
Implement a Color Filter
This involves creating a color filter, that is, filtering an object by its color. For example, you can show the camera an object of a certain color and have that object appear boxed in the image. To do this, you’ll need to threshold the image based on color. Perhaps a morphological filter could be applied to obtain a more precise representation of the object.
Edge Filters
An edge filter must be applied and displayed in the output image. This can be achieved using a Canny or Laplace filter. The Canny Edge Detector is an edge detection operator used in image processing to detect a wide range of edges in images. It is widely used for feature extraction and object detection due to its ability to identify sharp changes in intensity within an image, which correspond to the boundaries of objects. The Laplace Filter, also known as the Laplacian of Gaussian (LoG) filter, is a second-order derivative edge detection technique in image processing. Unlike the Sobel or Canny edge detectors, which detect edges by evaluating the first derivative (the rate of change), the Laplace filter detects edges by calculating the second derivative (the rate of change of the rate of change). This can help identify areas where the intensity changes sharply in the image.
Convolutions
The proposal is to apply a convolution to the image obtained from the camera. This convolution can be used to generate a smoothed image or to enhance the image.
Optical Flow
Optical flow must be calculated from the camera image, thus depicting the motion of the image. Optical flow is a technique used in computer vision and image processing to estimate the motion of objects between two consecutive frames in a video or image sequence. It calculates the apparent motion of pixel intensities, which helps in understanding the movement of objects or the camera itself.
OpenCV provides functions to calculate optical flow. Check this tutorial!
Some tips:
- Pixel intensities and the frame rate (FPS) can influence the accuracy of the flow estimation.
For a more advanced implementation, you can select good feature points and track only those specific pixels, as shown in the following example:
Corner Detector
A corner detector needs to be implemented. A Harris corner detector could be used. The Harris corner detector is a popular algorithm used in image processing to identify corner points in an image.
Hough Transform
The Hough transform must be applied to the image. The Hough Transform is a technique used in image processing and computer vision to detect simple shapes, such as lines, circles, and other parametric curves, in an image.
Hints
Simple thresholding, Adaptive thresholding, Otsu’s thresholding
Demonstrative video of the solution
Contributors
- Contributors: Jose María Cañas, Vanessa Fernández, Jessica Fernández, Lucía Lishan Chen Huang
- Maintained by Pankhuri Vanjani and Sakshay Mahna, Javier Izquierdo.
References
- https://www.geeksforgeeks.org/color-spaces-in-opencv-python/
- https://www.learnopencv.com/invisibility-cloak-using-color-detection-and-segmentation-with-opencv/
- https://www.learnopencv.com/color-spaces-in-opencv-cpp-python/
- https://opencv-python-tutroals.readthedocs.io/en/latest/index.html
- https://www.geeksforgeeks.org/python-visualizing-image-in-different-color-spaces/?ref=rp
- https://realpython.com/python-opencv-color-spaces/
- https://medium.com/@jijupax/connect-the-webcam-to-docker-on-mac-or-windows-51d894c44468